Distributed Prime Number Calculation Tutorial using Ratio1 Python SDK
Education
**Important Update:** This blogpost predates version 2.5 of the Ratio1 Python SDK (previously known as the Naeural Edge Protocol SDK), so some methods, calls, and processes may have changed. For the most accurate information, please consult our latest posts, videos, and tutorials. Currently, the SDK is listed on PyPI as `naeural_client`, but going forward, we plan to rename it to simply `ratio1`.
In this tutorial, we will explore how to calculate prime numbers both locally and on a distributed network using the Ratio1 Python SDK. We will walk through setting up the development environment, running the prime number calculation locally, and deploying the same task to multiple edge nodes in a distributed manner for improved performance.
Setup
Before we dive into the actual code, we need to ensure the proper setup of the development environment and SDK.
1. Install the SDK
To get started, install the Ratio1 Python SDK using pip:
2. Configure the Environment
You need to configure a .env
file that contains the necessary credentials and environment variables. You can use the provided example file as a template:
Make sure to fill in the required fields in the .env
file with appropriate values.
3. Manage Private Keys
For security, never publish sensitive information such as private keys. To experiment with this tutorial on a test network, you can use a provided test key. Alternatively, you can generate a new private key using the SDK, which will store it in your working directory.
If using a provided private key, copy the example file from tutorials/_example_pk_sdk.pem
to _local_cache/_data/
, renaming it to _pk_sdk.pem
.
Local Execution: Finding Prime Numbers
To start off, we will write a program that finds all 168 prime numbers between 1 and 1000. The code will run on a local machine and use multithreading to speed up the calculation.
Prime Number Generation Logic
Here is the Python code to generate prime numbers locally:
The method local_brute_force_prime_number_generator
generates a random sample of 20 numbers and checks each one to see if it is prime. The program leverages ThreadPoolExecutor
to parallelize the work across multiple threads.
We run this method repeatedly until we collect 168 unique prime numbers, as shown below:
In this code, we continuously compute new prime numbers and only add them to our list if they haven’t been discovered yet. The program prints progress every 50 iterations.
Remote Execution: Distributed Prime Number Calculation
While the local method works, it’s limited by the resources of a single machine. To speed up the process, we can distribute the work across multiple nodes using the Ratio1 network.
Adapting Code for Distributed Execution
To adapt the local prime number generator for remote execution, we need to make some adjustments to the code:
Connecting to the Network
Once the code is adapted for remote execution, we need to connect to the Ratio1 network to discover available edge nodes. We do this by creating a session and using the on_heartbeat
callback to list online nodes:
Sending a Task to a Node
Once we have identified an available node, we can send the prime number calculation task to it. This node will distribute the work to other nodes and collect the results.
Handling Partial Results
As nodes process the task, they will periodically send partial results. We need a callback function to handle these results:
Deploying the Job
We now create and deploy the distributed task using the Ratio1 API:
Completing the Job
Finally, we wait for the distributed task to finish and close the session:
Conclusion
In this tutorial, we demonstrated how to find prime numbers locally and then scale the task to a distributed network using Ratio1. By leveraging the power of edge nodes, we can significantly improve the performance of computationally expensive tasks such as prime number generation.
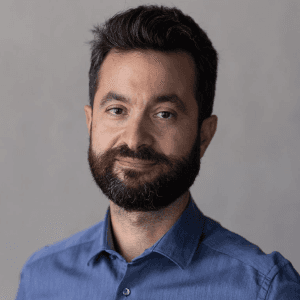
Andrei Ionut Damian
Dec 19, 2024