Building and Deploying a Custom Web Application using PyE2 SDK
Education
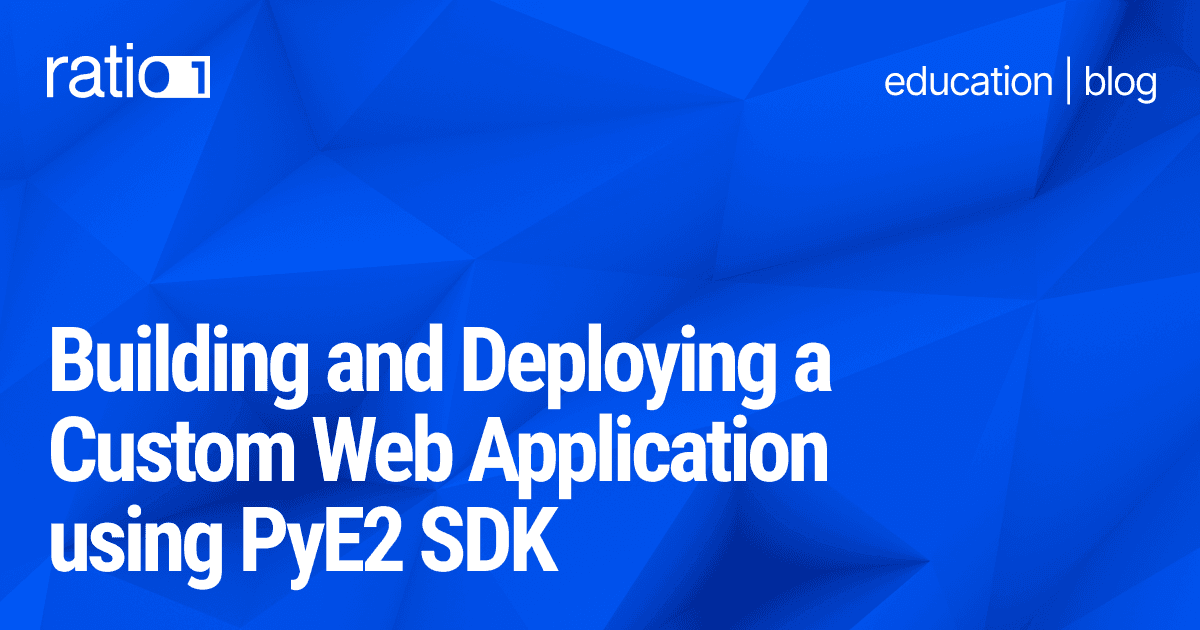
In this tutorial, we will explore how to set up and deploy a custom web application using the PyE2 SDK. We will create a FastAPI web app running on a local edge node, utilizing ngrok for exposing the server to the web. The web app will include several useful endpoints, such as a “Hello World” message, UUID generation, node address retrieval, and time series forecasting.
Setup
Before diving into the code, we need to ensure our environment is correctly set up. If you haven’t done so already, follow the steps below.
1. Install the SDK
You can install the PyE2 SDK by running the following command:
2. Configure the Environment
We need to set up a .env
file for network credentials. You can copy the example file provided:
Make sure to fill in the necessary details in the .env
file.
3. Manage Private Keys
Ensure that your private keys are securely managed. You can either generate a new key or use a provided test key as described in the previous tutorial. Make sure the key is placed in the correct directory (_local_cache/_data/
).
4. Obtain ngrok Token
To expose the local FastAPI server, you will need an ngrok account and an auth token. Visit ngrok’s website to create an account and retrieve your auth token. This is essential for making your web app accessible from the internet.
Building the Web App
In this tutorial, we will create a basic FastAPI web application that exposes several endpoints. These endpoints include:
A “Hello World” endpoint
An endpoint to generate UUIDs
An endpoint to retrieve the node address
A time series forecasting endpoint
Web App Code
Here is the complete Python code to set up and deploy the web app:
Key Functions
hello_world
Endpoint:
This is a simple “Hello World” endpoint that greets the user by name. The name is passed as a query parameter, and the response includes the node address from the PyE2 plugin.get_uuid
Endpoint:
This endpoint generates and returns a new UUID.get_addr
Endpoint:
This endpoint returns the address of the node running the web app.predict
Endpoint:
This is a time series forecasting endpoint that takes a series of integers and predicts future values based on the given number of steps.
Exposing the Web App via ngrok
The code uses ngrok
to expose the FastAPI server to the web. To enable this, ensure you have your ngrok_edge_label
and token ready.
Set your
node
variable to the address of the edge node you wish to use.Set your
ngrok_edge_label
with the label assigned to your ngrok account.
After that, the app can be deployed and accessed via the ngrok URL.
HTML Frontend
The application also serves a simple HTML frontend, which can be accessed via the root URL (/
). The HTML file is located at PyE2/tutorials/8. custom_code_fastapi_assets/index.html
and can be customized based on your needs.
Running the Application
Once the app is set up and endpoints are defined, we deploy it:
Finally, we start the session and let the web app run until explicitly closed:
The application will be accessible at the ngrok URL, where you can interact with the various endpoints.
Try the Forecasting Feature
You can also experiment with the time series forecasting feature directly by visiting the deployed version of this tutorial at https://naeural-013.ngrok.app/. This endpoint accepts a series of integers and predicts future values based on the number of steps specified. It’s a great way to test the power of the distributed time series forecasting on real-world data!
Conclusion
In this tutorial, we’ve walked through creating a simple FastAPI-based web application using the PyE2 SDK. This application provides several useful endpoints, including a basic “Hello World” message, UUID generation, node address retrieval, and time series forecasting.
By using ngrok, we expose the local edge node and make it accessible from anywhere. This setup can serve as a foundation for more advanced web applications deployed on distributed edge networ
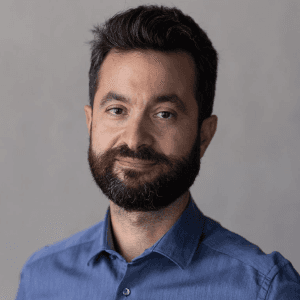
Andrei Ionut Damian
Dec 20, 2024
©Ratio1 2024. All rights reserved.